Following on from my first PIR sensor module article I thought I would create a Python script that allowed me to easily measure the reset time.
That way I could attach a module, run the script and measure the time it took for the output pin to drop back to the Low state. Then I could tweak the trimming resistor and adjust the reset time to my preferred value in a more controlled and precise way.
Example Python Script
The following script assumes you have your PIR module connected to the GPIO header as shown in Part 1. The example uses GPIO7 (Pin 26).
Cut and paste the script below into a text file and transfer to the Pi or download the script directly using this link (recommended).
#!/usr/bin/python #+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ #|R|a|s|p|b|e|r|r|y|P|i|-|S|p|y|.|c|o|.|u|k| #+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ # # pir_2.py # Measure the holding time of a PIR module # # Author : Matt Hawkins # Date : 20/02/2013 # Import required Python libraries import time import RPi.GPIO as GPIO # Use BCM GPIO references # instead of physical pin numbers GPIO.setmode(GPIO.BCM) # Define GPIO to use on Pi GPIO_PIR = 7 print "PIR Module Holding Time Test (CTRL-C to exit)" # Set pin as input GPIO.setup(GPIO_PIR,GPIO.IN) # Echo Current_State = 0 Previous_State = 0 try: print "Waiting for PIR to settle ..." # Loop until PIR output is 0 while GPIO.input(GPIO_PIR)==1: Current_State = 0 print " Ready" # Loop until users quits with CTRL-C while True : # Read PIR state Current_State = GPIO.input(GPIO_PIR) if Current_State==1 and Previous_State==0: # PIR is triggered start_time=time.time() print " Motion detected!" # Record previous state Previous_State=1 elif Current_State==0 and Previous_State==1: # PIR has returned to ready state stop_time=time.time() print " Ready ", elapsed_time=int(stop_time-start_time) print " (Elapsed time : " + str(elapsed_time) + " secs)" Previous_State=0 except KeyboardInterrupt: print " Quit" # Reset GPIO settings GPIO.cleanup()
Alternatively the script can also be easily downloaded onto your Pi directly using this command line :
wget https://www.raspberrypi-spy.co.uk/archive/python/pir_2.py
The script can then be run using :
sudo python pir_2.py
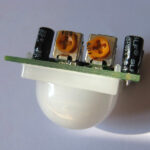
When run the script waits for the output pin to go Low. Just like in pir_1.py it prints a message to the screen when movement is detected. The main difference is that it now measures the elapse of time between the output pin going High and it returning to Low.
You can use a small screwdriver to tweak the “time” control to increase or decrease the time. Once you’ve triggered the module it is important to stay still so you don’t increase the time the output stays High which will mess up your results.
A half turn will result in the reset time being increased by a few minutes so it’s best to make adjustments in small increments.
are available on eBay.
6 Comments
Hi Matt,
Stumbled across your site while looking at circuit labs for pi circuits. Co-incidentaly, an equivalent PIR arrived in the post yesterday, so I’m testing it out with your code as we speak (albeit using the quick2wire interface board and their api – I’m new to electronice, so didn’t trust myself not to take out the Pi!).
How have you found the performance of your PIR – even when I’m sitting down out of sight, I seem to get a number of spurious readings from it – fairly steady stream of detections, alternating between 1 and 2 seconds.
Also, I guess this might be a Pi performance issue, but do you notice much of a lag between making a detectable movement and it registering – there seems a noticeable delay (I’m running agocontrol.com on the pi, but no gui).
Cheers
James
Hi Matt,
Have you ever tried using several PIR motion sensors? It seems to be workign well with 1 sensor, but when I tried to read from 2 ports one by one the code failed
It should work with 2 sensors as they can have separate GPIO pins. A lot of the code would need to be duplicated. It’s not a very efficient way of handling two sensors but should be possible.
Update on several sensors:
Tried with 2 PI’s:
1- Grove, first model of Raspberry pi + 4 motion sensors (given a large room) – didn’t work,
2- Grove+ Raspberry Pi 2 – did work.
I understand that it is not the most efficient ways to treat sensors as you have to force sleep after each sensor read and read sequentially but I have a large room with ceiling obstruction. What is the more efficient way to do it then?
A better technique would be to create a ‘class’ to define a PIR device. Then you use the class to create as many instances as required. This would reduce the code duplication.
Hi, i have a one cuestion: can it put vaious sensors in unique pin? Thanks